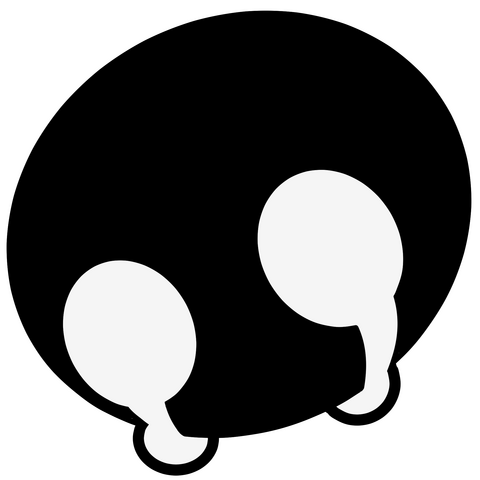
Restart Heart (18+ Visual Novel)
Brought to you by QueenLilithPrime and Fluorescent Red Studios
It is illegal and unethical for anyone below the age of 18 to interact with anything regarding this visual novel.
If you use this for your own code you don't have to credit me but I would appreciate it if you did!
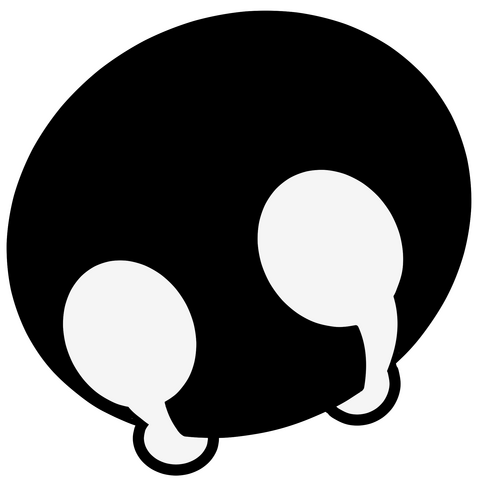
Coding Help from the Developer of Restart Heart
I won't gatekeep coding! Anything on this site feel free to adjust and use for your own purposes!
Just remember that all characters included are my own property and you cannot use my characters and their likeness for profit.I made this in order to help game developers, fans who are curious, or people who want to learn! Sometimes I couldn't find the answers for these things online or didn't realize how things worked until I did more research. A lot of code is out on the net without much explanation, so this will be a thoroughly explained tutorial site. Hopefully this helps!Much love,
Lilith
Resources
What do you use for coding?
Where did you get...
Image Gallery Code - ZeilLearnings
Kinetic Text Code - Wattson
Achievement Code - FeniksDev
How do you learn Ren'py?
Ren'py comes with a built-in tutorial game that gives you lots of code and examples you can use freely within your own game. You can also search in the Lemmasoft Forums for Ren'py help, and there's even a Ren'py Discord Server where you can ask for help on things. Ren'py Tom even has a website with more extensive explanations called Ren'py Documentation.
I also highly suggest learning the basics of Python, which is the coding language Ren'py is based on.
Characters and Player
How and why do you define your characters?
I'll go piece by piece to explain this!I use single/double letter definitions for characters in order to save myself time while writing!Color sets the color of the name whenever it appears on screen.Callback is what gives the voice blip noise.Style is something I use in order to make the names look a little fancier against the text box background.Image is important to use to set what the character sprite name is. I'll go over this further in sprites!I explain why I use two name variables further down!
Code Examples
define a = Character("[aa] (He/Him)", color="#99DDFF", callback=blip1, style="outlinesforeverybody", image="chris")define b = Character("[bb] (She/They)", color="#087762", callback=blip3, style="outlinesforeverybody2", image="bess")define c = Character("[cc] (They/Them)", color="#ffafaf", callback=blip2, style="outlinesforeverybody", image="sammy")define d = Character("[dd] (She/Her)", color="#FF4444", callback=blip3, style="outlinesforeverybody", image="blaire")define e = Character("[ee] (He/They)", color="#cf9fff", callback=blip1, style="outlinesforeverybody", image="ezra")define f = Character("[ff] (They/Them)", color="#3e4ad1", callback=blip2, style="outlinesforeverybody2", image="steph")define k = Character("[kk] (He/Him)", color="#B37A50", callback=blip1, style="outlinesforeverybody", image="kenneth")
define aa = "Chris"
define bb = "Bess"
define cc = "Sammy"
define dd = "Blaire"
define ee = "Ezra"
define ff = "Steph"
define kk = "Kenneth"
a "How are you feeling? Was your date with [ee] nice?"
How do you code the voice blips into the name?
I use callback code from Ren'py! I've adjusted it a bit from the original but I still use that code as the base!
There are four different variants used in Restart Heart for voice blips.
These voice blips are different pitches, and I usually use Blip 1 for masculine voices, Blip 2 for androgynous voices, and Blip 3 for feminine voices. Blip 4 is an amalgamation of the others and sounds glitchy when used.
Code Examples
init -2 python:
def blip1(event, interact=True, **kwargs):
if not interact:
return if event == "show":
renpy.sound.play("audio/blip1.ogg", loop=True) elif event == "slow_done" or event == "end":
renpy.sound.stop() def blip2(event, interact=True, **kwargs):
if not interact:
return if event == "show":
renpy.sound.play("audio/blip2.ogg", loop=True) elif event == "slow_done" or event == "end":
renpy.sound.stop() def blip3(event, interact=True, **kwargs):
if not interact:
return if event == "show":
renpy.sound.play("audio/blip3.ogg", loop=True) elif event == "slow_done" or event == "end":
renpy.sound.stop()
How do you set up player inputs?
There are quite a few ways do to player inputs for their names but this is how I prefer to do things!
Why do you use name.title()?
This makes sure that no matter how the player inputs their name (i.e. NAME, name, nAmE) it will always be properly capitalized! These are called python string methods!
.capitalize()
capitalizes the first letter of the string
.upper()
makes the entire string uppercase
.lower()
makes the entire string lowercase
.swapcase()
swaps all the lowercase to uppercase and all the uppercase to lowercase
.strip()
removes any leading spaces or trailing spaces
.title()
capitalizes the first letter of every word
Code Examples
define y = Character("[name] ([prn1!c]/[prn2!c])", whobold = True, color="#ffffff", callback=blip2)define z = Character("{size=-15}{i}Your Thoughts...{/size}{/i}", whatprefix="{i}{size=-5}", what_suffix="{/i}{/size}", color="#7a7a7a")
python:
name = renpy.input("What's my name again?") name = name.strip() or "Sugar" name = name.title()
What is the !c for?
It's called a conversion flag! You can use them in [ ]'s to adjust the variable.
!c
capitalizes the first letter
!u
makes it entirely uppercase
!l
makes it entirely lowercase
How do you do the name easter eggs?
This is one of the first things I tried to learn how to do in ren'py because I thought it was very fun to see in games!
All you have to do is use your name variable (I use name for simplicity) and use if statements!
You can see I can rename characters using my double letter variable, and this way their pronouns are unaffected.
I can also tease players who don't input any names! If you don't input and simply hit enter it'll automatically set your name to Sugar and includes an Easter Egg about Ezra naming you (This also occurs if you input your name as Sugar). If you input something along the lines of Name or Player you'll get another easter egg as shown to the right.
Code Examples
if name == "Ezra":
"There's already a character named Ezra, so... "
extend "their name is now Ezekiel."
$ ee = "Ezekiel"elif name == "Chris" or name == "Christopher":
"There's already a character named Chris, so... "
extend "their name is now Cole."
$ aa = "Cole"elif name == "Name" or name == "Y/n" or name == "Y/N" or name == "Yn" or name == "MC" or name == "Mc" or name == "Me" or name == "You" or name == "Player":
"fuck, really?... fine." "..." "Nope, I can't have you being named that." "Fuck it"
extend "... I'm renaming you." $ name = "Sugar"
Pronoun Code (and Genitalia Code for 18+ Games)
If you copy this please credit me on this, as it took me a lot of work to get this set up right!
How do you make custom pronouns?
So I wrote all of this pronoun code myself because other set-ups for pronouns didn't really make sense for me. I write RH primarily in second-person and I'm not great with grammar...
So all of this is what makes sense to me, it might not make sense to you but that's okay!
Each of the variables are as follows:
Variable Name | Generic | Feminine | Masculine |
---|---|---|---|
gender | nonbinary | woman | man |
title1 | partner | girlfriend | boyfriend |
title2 | spouse | wife | husband |
prn1 (subjective) | they | she | he |
prn2 (objective) | them | her | him |
prn3 (possessive) | their | her | his |
prn4 (reflexive) | themselves | herself | himself |
pronounstyle | plural | single | single |
prnst_is | are | is | is |
prnst_s | 're | 's | 's |
So how do you use these in text?
With the pronouns all you'd have to do is use them like other variables! I would suggest something like...
a "[prn1]'[prnst_s] an adult! [prn1] can think for [prn4]."
Which would spit out any of the below depending on the player
"He's an adult. He can think for himself"
"She's an adult! She can think for herself."
"They're an adult they can think for themselves."
I also have multiple pronouns in my game as an option for players to have more than one pronoun set in Restart Heart
Variable Name | Which variable it ties to | Variable Name | Which variable it ties to |
---|---|---|---|
multiprns | checks if player has >1 pronoun | ||
custprn1a | prn1 | custprn2a | prn2 |
custprn3a | prn3 | custprn4a | prn4 |
pronounstylea | pronounstyle | ||
custprn1b | prn1 | custprn2b | prn2 |
custprn3b | prn3 | custprn4b | prn4 |
pronounstyleb | pronounstyle | ||
custprn1c | prn1 | custprn2c | prn2 |
custprn3c | prn3 | custprn4c | prn4 |
pronounstylec | pronounstyle |
How does the multiple pronoun system work?
I use a bunch of label calls!
Code Examples
label prns4playertime:
if multiprns:
call expression multiprns
else:
pass
The above label I call whenever I need to use the players pronouns in order to randomize which one is picked.
I use call expression multiprns
in order to make it streamlined!
Calling multiprns
itself allows me to use whatever the variable is set to as a label. In order to make sure you check the variable rather than the word 'multiprns' you MUST use expression
with your call function.
Depending on what kind of pronouns the player choses, the multiprns
variable will change! Here's an example of what I set the variable to depending on the pronouns the player picks!
Pronouns Chosen | Multiprns variable string |
---|---|
Single Pronoun | False |
She/They | "she_they" |
He/They | "he_they" |
Any pronouns | "any_prn" |
Two Custom Pronouns | "customprns2" |
Three Custom Pronouns | "customprns3" |
Code Examples
Here's the code I use for the randomization below!
label she_they:
$ prnroll = renpy.random.randint(1,2) if prnroll == 1:
$ prn1 = "she"
$ prn2 = "her"
$ prn3 = "her"
$ prn4 = "herself"
$ pronounstyle = "single"
$ prnst_is = "is"
$ prnst_s = "s"
elif prnroll == 2:
$ prn1 = "they"
$ prn2 = "them"
$ prn3 = "their"
$ prn4 = "themselves"
$ pronounstyle = "plural"
$ prnst_is = "are"
$ prnst_s = "re" returnlabel he_they:
$ prnroll = renpy.random.randint(1,2) if prnroll == 1:
$ prn1 = "he"
$ prn2 = "him"
$ prn3 = "his"
$ prn4 = "himself"
$ pronounstyle = "single"
$ prnst_is = "is"
$ prnst_s = "s"
elif prnroll == 2:
$ prn1 = "they"
$ prn2 = "them"
$ prn3 = "their"
$ prn4 = "themselves"
$ pronounstyle = "plural"
$ prnst_is = "are"
$ prnst_s = "re" returnlabel any_prn:
$ prnroll = renpy.random.randint(1,3) if prnroll == 1:
$ prn1 = "she"
$ prn2 = "her"
$ prn3 = "her"
$ prn4 = "herself"
$ pronounstyle = "single"
$ prnst_is = "is"
$ prnst_s = "s"
elif prnroll == 2:
$ prn1 = "he"
$ prn2 = "him"
$ prn3 = "his"
$ prn4 = "himself"
$ pronounstyle = "single"
$ prnst_is = "is"
$ prnst_s = "s"
elif prnroll == 3:
$ prn1 = "they"
$ prn2 = "them"
$ prn3 = "their"
$ prn4 = "themselves"
$ pronounstyle = "plural"
$ prnst_is = "are"
$ prnst_s = "re" returnlabel customprns2:
$ prnroll = renpy.random.randint(1,2) if prnroll == 1:
$ prn1 = custprn1a
$ prn2 = custprn2a
$ prn3 = custprn3a
$ prn4 = custprn4a
$ pronounstyle = pronounstylea
if pronounstyle == "single":
$ prnst_is = "is"
$ prnst_s = "s"
else:
$ prnst_is = "are"
$ prnst_s = "re"
elif prnroll == 2:
$ prn1 = custprn1b
$ prn2 = custprn2b
$ prn3 = custprn3b
$ prn4 = custprn4b
$ pronounstyle = pronounstyleb
if pronounstyle == "single":
$ prnst_is = "is"
$ prnst_s = "s"
else:
$ prnst_is = "are"
$ prnst_s = "re" returnlabel customprns3:
$ prnroll = renpy.random.randint(1,3) if prnroll == 1:
$ prn1 = custprn1a
$ prn2 = custprn2a
$ prn3 = custprn3a
$ prn4 = custprn4a
$ pronounstyle = pronounstylea
if pronounstyle == "single":
$ prnst_is = "is"
$ prnst_s = "s"
else:
$ prnst_is = "are"
$ prnst_s = "re"
elif prnroll == 2:
$ prn1 = custprn1b
$ prn2 = custprn2b
$ prn3 = custprn3b
$ prn4 = custprn4b
$ pronounstyle = pronounstyleb
if pronounstyle == "single":
$ prnst_is = "is"
$ prnst_s = "s"
else:
$ prnst_is = "are"
$ prnst_s = "re"
elif prnroll == 3:
$ prn1 = custprn1c
$ prn2 = custprn2c
$ prn3 = custprn3c
$ prn4 = custprn4c
$ pronounstyle = pronounstylec
if pronounstyle == "single":
$ prnst_is = "is"
$ prnst_s = "s"
else:
$ prnst_is = "are"
$ prnst_s = "re" return
What about 18+ scenes?
Each of the 18+ variables are as follows:
Variable Name | Generic | Feminine | Masculine |
---|---|---|---|
nsfw | |||
genitalia | other/intersex | vagina | penis |
underwear | underwear | panties | boxers |
breasts | nipples | breasts | nipples |
chest | chest | breasts | chest |
praiseterm | |||
usespraiseterms |
So how do you use these in text?
Alrighty so with 18+ scenes you can either entirely make them 100% ungendered/sexed or make alternate texts for different types and scenes.
I personally have a hard time writing scenes with descriptions when I have to leave things out, so I go the long way and write seperate scenes for different genitalia. However, I still use the other variables within those same scenes.
"You looked at your own [chest], then to the [underwear] you were wearing."
Which would spit out any of the below depending on the player
"You looked at your own chest, then to the boxers you were wearing. "
"You looked at your own breasts, then to the panties you were wearing."
"You looked at your own chest, then to the underwear you were wearing."
if usespraiseterms:
"Hey~ Aren't you such a [praiseterm]?~"
else:
"Hey~ You're such a [praiseterm]~"
Which would spit out any of the below depending on the player
"Hey~ Aren't you such a good girl?~"
"Hey~ Aren't you such a good boy?~"
"Hey~ You're such a good slut~"
Code Examples
label nsfwexamplescene:
if not nsfw:
"You slept with the character."
else:
# Here is where you would put the smut in for the character if genitalia == "vagina":
# Here is where you would write vagina specific smut
elif genitalia == "penis":
# Here is where you would write penis specific smut
elif genitalia == "intersex":
# Here is where you would write vagina + penis smut
elif genitalia == "other":
# Here is where you would write non-specific smut # More smut goes here!
What about 18+ scenes?
Remember when you're writing 18+ scenes, especially in VN's where people are meant to have a self-insert they are playing as, that you should always take the utmost care in handling intimate scenes and gender/identity.
Many players who are trans, non-binary, or gay often struggle to see representation within media and want to use these medias in the same way others do, as an escape. So please take care to listen to feedback from players and always be inclusive in your works.
Players shouldn't have to misgender themselves or deal with misgendering or uncomfortable gender/sex scenes simply because of they don't have any choice.
If you have made it this far, I'm assuming you plan to use the pronoun selection and possibly the genitalia selection I have coded. So please listen when I say that it's important that the player character be someone they can insert themselves into.
If you have a preset character that the player will play as, that's perfectly fine as long as people are aware of that ahead of time, especially in dating games. However, if you want a player to be able to self-insert you need to not assign attributes to them.
All MC's will have their own personality simply because of the nature of writing a story, even for self-inserts. But you can't assign traits like straight hair, impose a skin tone, weight or height, or assume the sex/gender of your players.
Players don't want to experience dysphoria when playing a game, so you need to keep that in mind. Especially when writing 18+ scenes. Many players may lose interest in your product if they are forced into a binary/sex that they are not a part of.
Obviously we all have our own demographics that we are pandering to, but in games where you can be inclusive, you should.
Ramble over, sorry lmao.
Sprites
My method for Sprites
When making sprites, the best way for expanding choices and options for yourself in the future development of a game is to have all of the parts separated.
There should be separate images for Eyes, Mouth, Brows and other Facial/Expressive Indicators like Sweat, Blush, or Anger symbols.
Noses can usually be kept on the base sprite as they usually don't move around the face, but if your art style does have noses move, you can add those as well!
When doing expressions and adding the additions like blushing or sweat or other markers of expression there are a few ways you can do it but I would suggest blush being on the bottom and other expression parts to be on top, with the additives above all the others.
For the base sprites that have no face, can break them up even further in order to have layers that allow for different hairstyles, outfits, body states, and more! This will allow for easy additions as well.
This also allows for smaller file size so the game doesn't take up as much space on the players device.
What should you use for sizing?
I personally use images that are the following sizes
640x1080 for games that are 1920x1080
1280x2160 for games that are 3840x2160You can either export images this size or work on a canvas this size.
I personally work on a larger canvas (1280x2160) and export smaller.
Why are some images named with spaces and others named with underscore's?
The images named with spaces are images that use ren'py built in tagging function, that allows you to use the show/hide function to its fullest! Further information on it can be found here.
Why do you use Layered Image instead of something else?
I use layeredimage for it's versatility and the fact it is far easier to add things to than anything else I've used in Ren'py. I have tried many different ways and this one always ends up the best for me.With layeredimage you not only optimize your game files and cut down on coding but you also give yourself more customization for features!
How do I get started?
UNDER CONSTRUCTION COMING SOON
Code Examples
Animating
image ezra_eyes_direct:
"ezra/eyesdirect.png"
choice:
4.5
choice:
3.5
choice:
1.5
"ezra/eyesclosed.png"
.25
repeat
UNDER CONSTRUCTION COMING SOON
What does choice do and why is it used in animating?
It randomizes the length from the chosen lengths you put! So the blinks in that example can be between 4.5, 3.5, and 1.5 seconds apart
What does the .25 at the end mean?
How long the final image is held for.
Contact Form
Creator and Developer
Queen Lilith Prime
You are allowed to create fan-content for Restart Heart however you cannot use my characters or universe for profit.What does this mean?
I don't mind if artists do commissions of my characters if requested by their client, however selling merchandise of my game and it's characters is not allowed.Please remember I am an indie game developer and official merchandise for Restart Heart is available!